How to Load Test Web API in just 5 minutes without any testing tool
The Problem
Testing REST (Representational State Transfer) Api is very common task for any organization. Ideally, dedicated test team or automation runs to do api test after development. But during development most of the time developer test with small set of data. What if we want to test for massive data, like millions of request? This can be easily achieve in less than 5 min without any special test tool.
Design your Api’s to handle load during peak hours, so performing load test is essential. For instance, 10000 requests/minute or 200 requests/second can generate millions of request load on server.
The Solution
To perform load test, we have to use some automated load tools. There are several free/paid tools available. These tools comes with lot of great features which involve learning time and cost.
What if we can achieve the purpose without using any specific testing tools? Yes, we can do it very easily in any programming language. I am showing example using C#.
Save every single penny wherever possible.
– General thought
To load test, rationale is very simple –
Send thousands of web requests simultaneously.
– Millions of Web Request
How to implement the solution
We can do this in simple C# console App or Linqpad (great tool for .Net developer) with HttpClient in just 5 minutes. I am using Linqpad to run this script.
void Main() { System.Threading.Tasks.Task.Run(async () => { "Start Generating Load".Dump(); await RunTest(); "Completed.".Dump(); }); } public async System.Threading.Tasks.Task RunTest() { int MAX_ITERATIONS = 500; int MAX_PARALLEL_REQUESTS = 500; int DELAY = 100; // Collection of Url's to test. Change to your valid Url's. string[] urls = new string[] { "https://hostname.azurewebsites.net/api/datatest", "https://hostname.azurewebsites.net/api/datatest/add" }; using (var httpClient = new System.Net.Http.HttpClient()) { // To add any headers like Bearer Token, Media Type etc. //httpClient.DefaultRequestHeaders.Add("Authorization", $"Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.xxxxxx"); for (var step = 1; step < MAX_ITERATIONS; step++) { $"Started iteration: {step}".Dump(); var tasks = new List<System.Threading.Tasks.Task<System.Net.Http.HttpResponseMessage>>(); for (int i = 0; i < MAX_PARALLEL_REQUESTS; i++) { tasks.Add(httpClient.PostAsync(urls[i % 2], null)); } // Run all 300 tasks in parallel var result = await System.Threading.Tasks.Task.WhenAll(tasks); $"Completed Iteration: {step}".Dump(); // Some delay before new iteration await System.Threading.Tasks.Task.Delay(DELAY); } } }
I ran this script for 500 iterations from 300 to 1000 parallel request per iteration for few hours. The web api was developed on .Net Core 3.1, hosted on Azure Linux Web App B1 plan. You can look for another post on why to host on linux web app over windows – Deploy ASP.NET Core apps to Azure App Service with lesser cost , which is why I chose Linux Web App over Windows. The test script was able to generate 2 millions of requests successfully from my Surface Book within few hours.
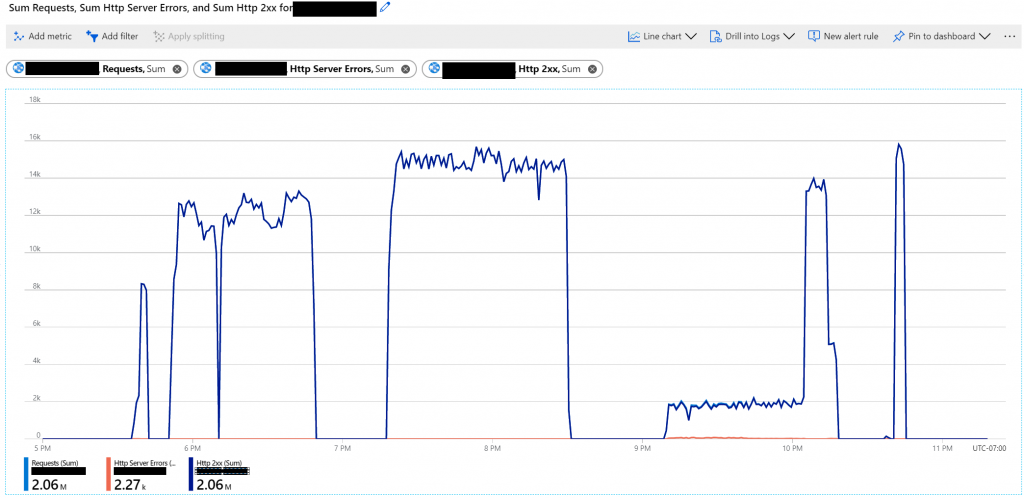
Conclusion
To summarize, we should always test our code for performance, scale, which results into better quality code.
I hope you found this post helpful.